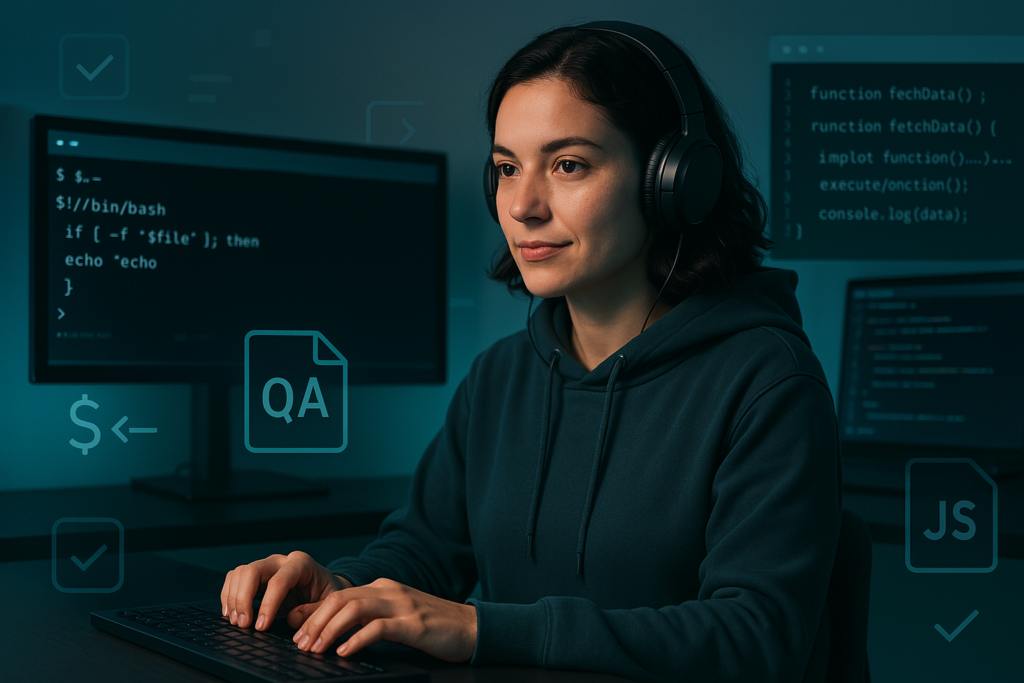
Intro:
Some testers think automation starts with Selenium or Cypress. Others think you’re not technical unless you’re pushing code to CI/CD. Here’s the truth: most testing bottlenecks are not because you lack a framework—they happen because you don’t know how to script around the problem.
Knowing how to use Bash, Node.js, and CLI tools can completely change the way you test—whether you’re manual or automated. You’ll run faster tests, debug smarter, and even impress devs who realize you’re not just “clicking around.”
Let’s break it down.
What Does Scripting Even Mean for QA?
Scripting isn’t just writing big automation test suites. It’s about solving small, repeatable pain points like:
- Cleaning up a test data file
- Creating hundreds of test users
- Parsing an API response
- Hitting an endpoint before a UI test
- Searching through logs to find a failure
In short? It’s using small scripts to save time and eliminate repetitive, error-prone manual work.
Learn to Crawl: How to Run a Script for the First Time
Before you start looping through files or chaining API calls, you need to get comfortable running a basic script from your terminal.
🐚 Running Your First Bash Script
- Open a terminal (Command Prompt, Git Bash, Terminal, or WSL)
- Create a file called
hello.sh
- Paste this in:
echo "Hello, QA World!"
- Save it
- Run it:
bash hello.sh
That’s it – you just ran your first script.
If you’re using Windows, install Git for Windows so you can use Bash commands with Git Bash.
🟦 Running Your First Node Script
- Install Node.js (LTS version)
- Create a file called
hello.js
- Paste this:
console.log("Hello from Node!");
- Run it:
node hello.js
Boom—you’ve now executed JavaScript outside the browser.
Don’t worry about complex setups. Just build the muscle memory first.
Why Bash Should Be in Your QA Toolbox
Bash is the command-line language for most UNIX/Linux systems (also works in WSL or Mac). Even Windows users benefit from Git Bash or PowerShell.
Here’s what you can do with Bash as a tester:
🔹 Loop Through Test Files
for f in ./data/*.json; do
echo "Validating $f"
jq . "$f"
done
This validates all JSON test data in a folder.
🔹 Download & Save API Responses
curl -s https://api.testapp.dev/users > users.json
You now have a mock dataset for local testing.
🔹 Check for 404s in URLs
while read url; do
code=$(curl -o /dev/null -s -w "%{http_code}" "$url")
echo "$url returned $code"
done < urls.txt
Great for link audits or broken environments.
🔹 Grep Logs for Error Tracing
grep -i "error" ./logs/app.log | tail -n 10
Find the last 10 error logs instantly.
Node.js for Testers Who Want More Power
Node.js isn’t just for devs. It’s also great for testers who:
- Need to write helper scripts
- Mock or hit APIs
- Transform JSON, CSV, or Excel files
- Interact with test runners (like Cypress or Playwright)
You don’t need to build a server. You just need to write utilities.
🔸 Read and Modify Test Data
const fs = require('fs');
const data = JSON.parse(fs.readFileSync('users.json', 'utf8'));
data.forEach(user => {
console.log(`Test user: ${user.name}`);
});
🔸 Generate Fake Data (with Faker.js)
const { faker } = require('@faker-js/faker');
for (let i = 0; i < 5; i++) {
console.log(faker.internet.email());
}
Perfect for registration, login, or spam flow testing.
🔸 Chain APIs Together
const axios = require('axios');
(async () => {
const res = await axios.post('https://api.site.dev/login', {
username: 'qa@test.com',
password: 'password123'
});
console.log(res.data.token);
})();
You can use that token to test other authenticated endpoints.
But I’m a Manual Tester—Why Bother?
Because these scripts will:
- Speed up your bug reproduction steps
- Let you trigger state changes faster than UI
- Help you dig deeper when something breaks
- Prove to your devs you understand what’s going on
- Let you create cleaner bug reports (with logs, tokens, evidence)
Most automation engineers aren’t better testers—they just have better tools. These are the tools.
You don’t need to become a full-time engineer. You just need to get dangerous enough to script your way around blockers.
How to Start Without Overwhelm
Start with one use case. Pick a task you hate repeating. Then:
- Try to do it manually in the terminal (curl, grep, etc.)
- Convert it into a Bash script (save it as
.sh
) - Later, rebuild it in Node if it needs logic or structure
- Save and document your scripts in a folder or your repo
Here’s a real-world example:
- Manual tester logs in to test site → uses browser dev tools to get auth token → pastes into Postman manually.
With a Bash+Node combo:
- You hit a login endpoint, capture token, inject it into test flow
- Save minutes per test session
Final Thoughts
This isn’t about replacing your tools. It’s about making YOU faster, smarter, and less dependent on bloated manual steps.
Learn just enough Bash and Node to:
- Script test flows
- Extract useful data
- Automate boring setups
No frameworks. No CI/CD. Just power at your fingertips.
We’ll build and publish reusable scripts soon on GitHub for our Playground. But you can start writing your own now.
Start small. Script something stupid. Then do it again.
This is a follow up post for Essential QA Tools for Website Testing – What Every Tester Should Be Using.