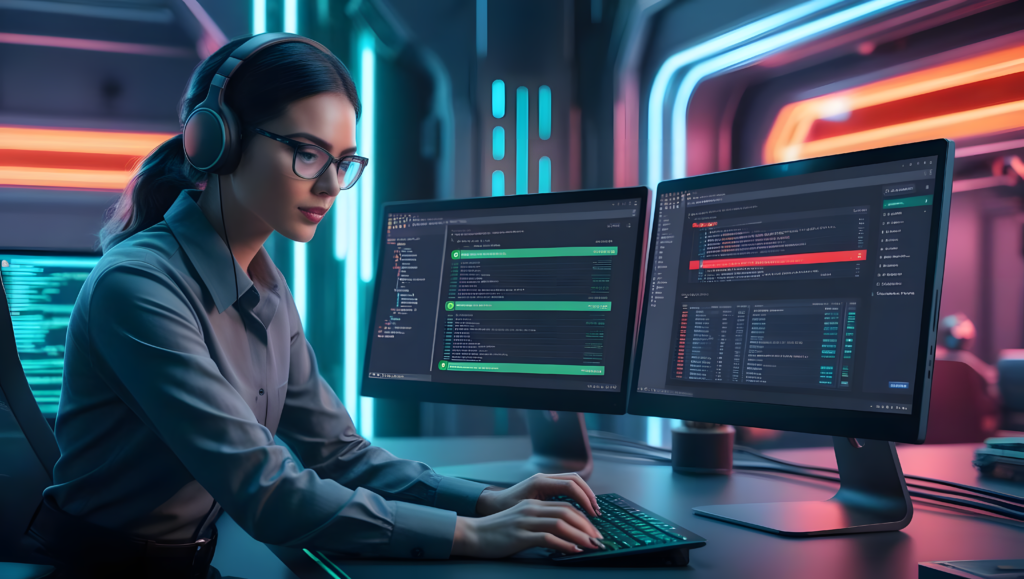
Introduction: Taking Cypress Beyond the Basics
Cypress is often praised for its simplicity and speed in UI automation, but many testers don’t realize its full potential. Beyond traditional end-to-end tests, Cypress can be used for API testing, network stubbing, component testing, accessibility validation, and even backend interactions. So here are some Cypress Automation testing tips for you.
Why You Should Go Beyond Basic Cypress Tests
Most testers start with Cypress by writing simple UI tests—filling forms, clicking buttons, and verifying text. While that’s great for beginners, real power comes when you unlock Cypress’s advanced features.
- Ever struggled with flaky tests? Cypress can help stabilize them.
- Tired of waiting for real API responses? Cypress lets you mock them.
- Want deeper insights into your app? Cypress allows network monitoring, performance analysis, and more.
This guide takes you beyond the basics, showing how to write smarter, more reliable Cypress tests and how to integrate these advanced techniques into your daily testing workflow.
📌 Want to test these techniques live? Head over to QA Testing Playground and fork the Cypress test suite to try them yourself!
1️⃣ Intercepting API Calls with cy.intercept()
Modern applications rely heavily on API calls to fetch and update data. What happens if your backend is down or slow? Cypress makes it incredibly easy to intercept, mock, and modify API requests, helping you isolate front-end behavior.
🔥 Use Case: Testing API Responses Without Backend Dependence
Let’s say you want to test how your app handles slow-loading API responses. Instead of relying on an actual delayed backend, we simulate the delay directly in Cypress.
Example Test: Simulating Network Delay
cy.intercept('GET', '/api/users', (req) => {
req.reply((res) => {
res.delay(3000); // Simulate 3-second delay
res.send({ users: [] });
});
});
cy.visit('/network-delay/');
cy.get('#loading-indicator').should('be.visible');
cy.wait(3000);
cy.get('#loading-indicator').should('not.exist');
📌 Test it live on: Network Delay Test
2️⃣ Handling Shadow DOM & Web Components
Modern front-end frameworks use Shadow DOM to encapsulate styles and behavior. The problem? Traditional selectors don’t work. Cypress provides built-in support to easily navigate and test these elements.
🔥 Use Case: Interacting with Shadow DOM Elements
cy.get('custom-element').shadow().find('.button').click();
📌 Test it live on: Dynamic Elements
3️⃣ Cypress for Backend & Database Validation
UI tests are great, but sometimes you need to verify that the data was actually saved in the database. Instead of switching between UI and SQL queries, Cypress allows you to interact with the database directly using cy.task()
.
🔥 Use Case: Checking Database Entries After Form Submission
cy.task('queryDb', 'SELECT * FROM users WHERE email = "test@example.com"').then((result) => {
expect(result).to.have.length(1);
});
📌 Test it live on: Form Testing
4️⃣ Cross-Browser & Multi-Domain Testing
By default, Cypress runs only in Chrome-based browsers, but with plugins like cypress-electron
, cypress-firefox
, and cypress-edge
, you can expand coverage.
📌 Test it live on: IFrame Handling
5️⃣ Cypress for Accessibility Testing (a11y)
Ensuring apps meet WCAG compliance is critical. Cypress, combined with cypress-axe
, can automatically detect accessibility issues and help teams ship inclusive products.
🔥 Use Case: Running Automated Accessibility Checks
import 'cypress-axe';
cy.injectAxe();
cy.checkA11y();
📌 Test it live on: Basic UI Testing
6️⃣ Advanced IFrame Handling in Cypress
Unlike Selenium, Cypress does not support cross-origin iframes easily. But using .then()
and cy.window()
, you can still interact with them.
🔥 Use Case: Testing Interactions Inside IFrames
cy.get('iframe').then(($iframe) => {
const body = $iframe.contents().find('body');
cy.wrap(body).find('#button').click();
});
📌 Test it live on: IFrame Testing
Conclusion: Leveling Up Your Cypress Tests
Cypress is much more than just a Selenium alternative. With network interception, database interactions, accessibility testing, and iframe handling, you can unlock highly advanced testing capabilities that most testers aren’t utilizing.
How to Get the Most Out of Cypress
1️⃣ Start integrating these techniques gradually. Don’t just use Cypress for UI—bring in API and backend validation.
2️⃣ Combine Cypress with other testing tools. Playwright, Jest, and Postman can complement Cypress for a full-stack test strategy.
3️⃣ Leverage CI/CD automation. Integrate Cypress with GitHub Actions or Jenkins to continuously test and deploy.
🚀 Next Steps:
- Fork the repo & run tests: Cypress Test Repo
- Practice real tests: QA Testing Playground
- Read more: QAJourney Blog
📌 Ready to automate? Start testing smarter today! 🚀